Gradleとは何者?インストール方法〜使い方までわかりやすく解説
Javaプログラミング学習をするうえで、「文字列を分割するには?」と悩んでいる方も多いのではないでしょうか?
「splitメソッドの使い方は?」
「特定の文字を使って文字列を分割する方法は?」
といった疑問に対して、本記事ではsplitメソッドについて初心者エンジニア向けに詳しく解説していきます。
※本記事で紹介するサンプルコードは、Java18で動作確認しています。
1.Javaのsplitメソッドとは?
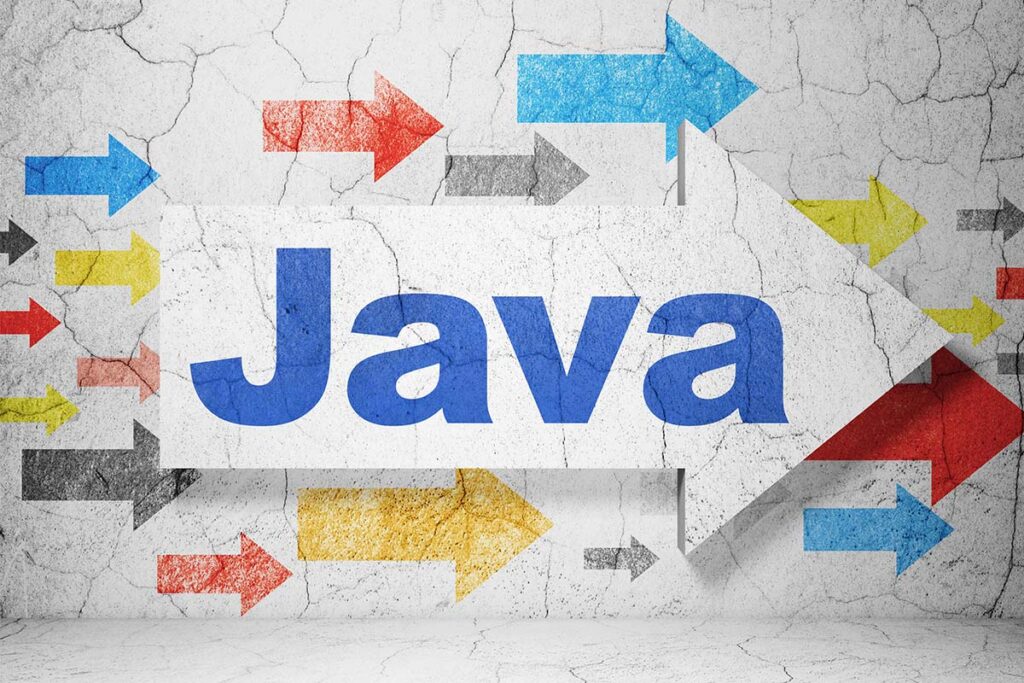
splitメソッドとは、ある文字列を、指定した区切り文字によって分割し、分割した文字列を配列として格納するメソッドです。
splitメソッドの基本書式は次の通りです。
String型変数名.split(区切り文字,分割回数);
第1引数の区切り文字には“,(カンマ)”や”;(セミコロン)”のほか、空白やタブ、改行を表す正規表現も設定できます。第2引数の分割回数を設定すると分割数を制御できます。第2引数は省略することも可能です。
2.splitメソッドの基本的な使い方
ここでは、splitメソッドの基本的な使い方を解説します。
①区切り文字を指定して分割する
さまざまな区切り文字を指定して分割するサンプルコードを見ていきましょう。
カンマで分割する
カンマで文字列を分割するには、第1引数に”,(カンマ)”を指定します。
サンプルコードを見てみましょう。
public class App {
public static void main(String[] args) {
String str = "one,two,three";
System.out.println(str);
String[] nums = str.split(",");
for (int i = 0; i < nums.length; i++) {
System.out.println(nums[i]);
}
}
}
実行結果:
one,two,three
one
two
three
カンマで分割する方法は、csvファイルを読み込む時などによく利用されます。
改行コードで分割する
改行コードで分割するには、第1引数に” \n”を指定します。
public class App {
public static void main(String[] args) {
String str = "one\ntwo\nthree";
System.out.println(str);
String[] nums = str.split("\n");
for (int i = 0; i < nums.length; i++) {
System.out.println(nums[i]);
}
}
}
実行結果:
one
two
three
one
two
three
空白で分割する
空白(半角スペース)で分割するには、第1引数に” ”を指定するのが簡単です。
public class App {
public static void main(String[] args) {
String str = "one two three";
System.out.println(str);
String[] nums = str.split(" ");
for (int i = 0; i < nums.length; i++) {
System.out.println(nums[i]);
}
}
}
実行結果:
one two three
one
two
three
もしくは、正規表現を使っても分割できます。その場合は、第1引数に”\s”を指定します。
public class App {
public static void main(String[] args) {
String str = "one two three";
System.out.println(str);
String[] nums = str.split("\s");
for (int i = 0; i < nums.length; i++) {
System.out.println(nums[i]);
}
}
}
実行結果:
one two three
one
two
three
どちらの方法を採用しても、同じ結果になることがわかりますね。
ピリオドで分割する
ピリオドで分割する場合、第1引数に”.(ピリオド)”を指定しても分割できません。これは、ピリオドが正規表現で利用される文字だからです。
正規表現ではピリオドは「任意の1文字」を表します。ピリオドとして扱うためには、第1引数に”.\\.”を指定します。
public class App {
public static void main(String[] args) {
String str = "one.two.three";
System.out.println(str);
String[] nums = str.split("\\.");
for (int i = 0; i < nums.length; i++) {
System.out.println(nums[i]);
}
}
}
実行結果:
one.two.three
one
two
three
このように特殊文字を使って分割する場合、エスケープ処理が必要になります。特殊文字の前に、” \\”を入れるとエスケープされます。
②分割回数を指定して分割する
splitメソッドの第2引数に1以上の整数を指定すると、分割数を制御できます。
サンプルコードを見ていきましょう。
public class App {
public static void main(String[] args) {
String str = "one,two,three,four,five";
String[] nums = str.split(",",4);
for (int i = 0; i < nums.length; i++) {
System.out.println(nums[i]);
}
}
}
実行結果:
one
two
three
four,five
分割回数を4に指定しているので、分割結果が4行になっているのが、わかりますね。
3.splitメソッドの応用的な使い方
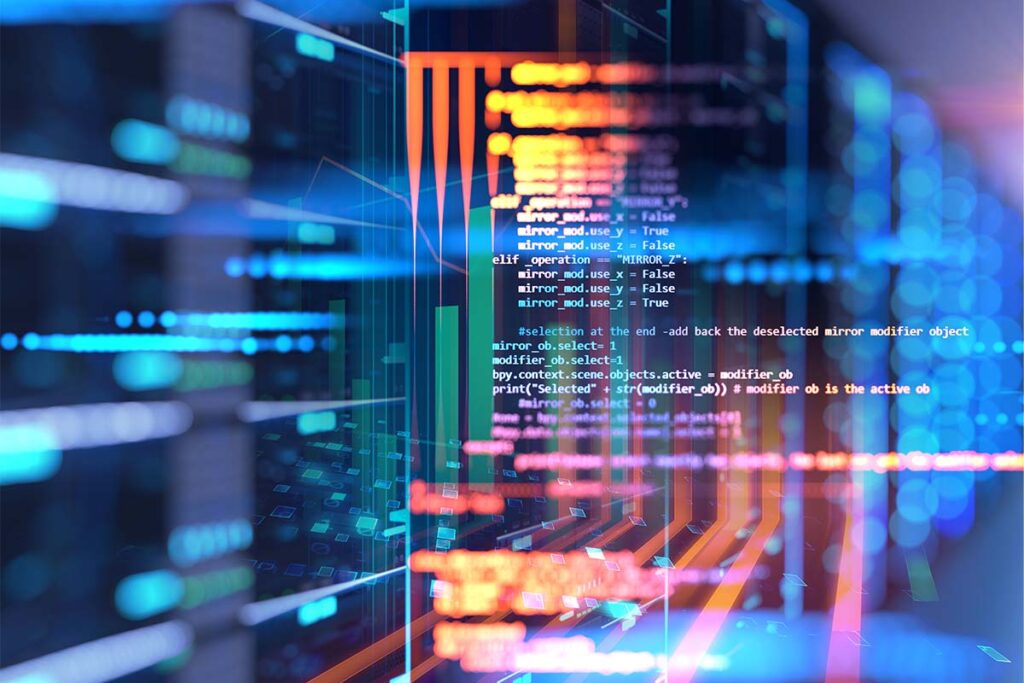
ここでは、正規表現や複数の区切り文字を使用して分割するなど、splitメソッドの応用的な使い方を紹介します。
①正規表現を使って分割する
splitメソッドの第1引数には正規表現を指定できます。サンプルコードを確認してみましょう。
public class App {
public static void main(String[] args) {
String str = "one,two*three;four,five";
System.out.println(str);
String[] nums = str.split("[,*]");
for (int i = 0; i < nums.length; i++) {
System.out.println(nums[i]);
}
}
}
実行結果:
one,two*three;four,five
one
two
three;four
five
”[]”は、正規表現では、「角括弧内の任意の1文字にマッチする」を意味します。今回のサンプルコードでは、”[]”内に含まれている”,” “*” で、文字列を分割します。
②間の空白文字を除外して分割する
間の空白文字を除外して分割する場合、正規表現を使用すると空白文字を除外して分割できます。
public class App {
public static void main(String[] args) {
String str = "one ,two ,three ,four ,five";
System.out.println(str);
String[] nums = str.split("[\s]*,");
for (int i = 0; i < nums.length; i++) {
System.out.println(nums[i] + " 長さ:" + nums[i].length());
}
}
}
実行結果:
one ,two ,three ,four ,five
one 長さ:3
two 長さ:3
three 長さ:5
four 長さ:4
five 長さ:4
第1引数に正規表現”[\s]*,”を指定すると、間の空白が除外されます。
③複数の区切り文字を指定して分割する
正規表現を利用すると、複数の区切り文字を指定できます。
サンプルコードを確認しましょう。
public class App {
public static void main(String[] args) {
String str = "one,two*three;four@five";
System.out.println(str);
String[] nums = str.split("[,*;@]");
for (int i = 0; i < nums.length; i++) {
System.out.println(nums[i]);
}
}
}
実行結果:
one,two*three;four@five
one
two
three
four
five
このサンプルコードでは、”,” ”*” ”;” ”@”の4つを指定して分割しています。
④空文字を指定して1文字ずつ分割する
第1引数に空文字を指定すると、1文字ずつ分割できます。
public class App {
public static void main(String[] args) {
String str = "one,two,three";
System.out.println(str);
String[] nums = str.split("");
for (int i = 0; i < nums.length; i++) {
System.out.println(nums[i]);
}
}
}
実行結果:
one,two,three
o
n
e
,
t
w
o
,
t
h
r
e
e
⑤末尾の空文字を除外しない
splitメソッドの第2引数を省略すると、末尾に空文字があった場合は省略されます。末尾の空文字を除去しない場合、第1引数にマイナス値を指定すると、空文字を含めた分割が行われます。
今回のサンプルコードでは、第2引数に”-1”を指定してみましょう。
public class App {
public static void main(String[] args) {
String str = "one,two,three,four,five,,";
System.out.println(str);
String[] nums = str.split(",",-1);
for (int i = 0; i < nums.length; i++) {
System.out.println(i+1 + ": " + nums[i]);
}
System.out.println("分割数:" + nums.length);
}
}
実行結果:
one,two,three,four,five,,
1: one
2: two
3: three
4: four
5: five
6:
7:
分割数:7
実行結果から、分割数が7で、空文字が除去されていないことがわかりますね。
⑥分割結果をListで取得する
通常、splitメソッドで分割した結果は配列に格納されます。配列ではなくListで取得したい場合は、分割した後にArraysクラスのasListメソッドを使用します。
import java.util.Arrays;
import java.util.List;
public class App {
public static void main(String[] args) {
String str = "one,two,three,four,five";
System.out.println(str);
List<String> nums = Arrays.asList(str.split(","));
for (String num : nums){
System.out.println(num);
}
}
}
実行結果:
one,two,three,four,five
one
two
three
four
five
4.splitメソッドを使う際の注意点
ここではsplitメソッドを使う際の注意点を解説します。
①対象文字列がnullの場合は例外が発生する
splitメソッドは対象文字列がnullの場合、例外「NullPointerException」が発生します。splitメソッドを使用する前にnullチェックを行うか、try-catch文で例外処理を行うのが良いでしょう。
今回のサンプルコードでは、nullチェックを行なっています。
public class App {
public static void main(String[] args) {
String str = null;
if(str != null) {
String[] nums = str.split(",");
for (int i = 0; i < nums.length; i++) {
System.out.println(nums[i]);
}
} else {
System.out.println("対象の文字列がnullです。");
}
}
}
実行結果:
対象の文字列がnullです。
②正規表現で特殊文字を使う場合はエスケープが必要
先述の通り、第1引数に特殊文字を使う場合はエスケープが必要です。”*”のような特殊文字を使う場合もエスケープしましょう。
import java.util.Arrays;
import java.util.List;
public class App {
public static void main(String[] args) {
String str = "one*two*three";
System.out.println(str);
List<String> nums = Arrays.asList(str.split("\\*"));
for (String num : nums){
System.out.println(num);
}
}
}
実行結果:
one*two*three
one
two
three